Coding Techniques Used
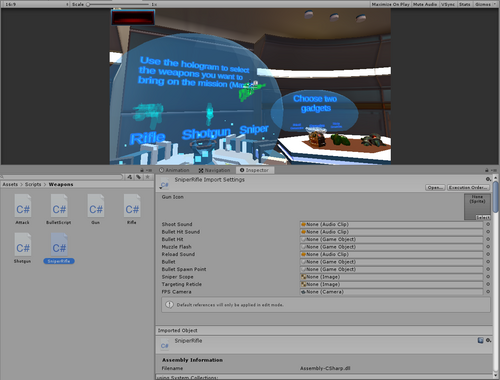
I tried to use programming patterns wherever possible in order to maximise re-usability and readability.
Inheritance Patterns
I used inheritance primarily for the enemy AI. I had a single Enemy class which would contain everything that was common between all enemies such as health, movement speed and weapons. Each type of enemy would then have a separate class which would inherit from the Enemy base class and the only real difference would be the behaviour of the enemy
Another example of when I used inheritance is for the weapons and gadgets (Image 1). For the weapons, I started with a simple rifle class which had all the attributes a gun could need such as ammo, ammo capacity, damage, accuracy, range, rate of fire, bullet model, bullet spawn point and muzzle flash particles, all of which could be easily edited in order to create multiple types of rifles. All that was then needed to create vastly different types of guns such as a shotgun was to create a new shotgun class which inherited from the rifle class and then override the shoot method which made it shoot multiple pellets instead of a single bullet like the rifle. Using the inheritance pattern for the guns and gadgets reduced the amount of duplicate code because instead of having multiple scripts where everything except one or two small things was different, it would be a single class and then multiple child classes that would only change what needed to be different. The whole point of using this pattern was to make it super simple to create new types of guns and gadgets with minimal effort.
Various interfaces were also used. E.g. IHurtable was implemented by anything that could take damage so that whenever a bullet or explosion hit something, it would simply check if what it collided with had the IHurtable interface and then call it's TakeDamage() function.
A similar thing was done for anything that could be interacted with. All intractable objects implemented the IInteractable interface and whenever they came into the interaction camera's frustum (This was a second camera attached to the main camera which had a more refined frustum), they would get the "e to interact" icon to show appear. When the user pressed "e" while looking at one of these objects, that would trigger the InteractWith() function which would usually then invoke a Unity Event. Unity Events were used to maximise customizability and re-usability.
Singleton:
The singleton was used numerous times as a way of getting an instance of an object easily an efficiently when that object was the only instance of that object in that scene. It was mostly used for controllers for things like the UI, the player, and the objective controller which were all things that there was only ever one of and were regularly accessed by other classes
State Pattern:
This pattern was primarily used for the AI. I used a switch enumerator to manage the AI's various states. These states were for behaviour such as idle, wandering, patrolling, attacking, retreating and evading and these states would only trigger under certain circumstances e.g. Attack or evade states could only be triggered when an enemy was within the AI's line of sight; the AI could only retreat if it's health was below a certain amount
Get Sci-Fi Shooter
Sci-Fi Shooter
Status | Prototype |
Author | Manning1999 |
Genre | Shooter |
Tags | 3D, Sci-fi |
More posts
- Initial DevelopmentNov 04, 2019
Leave a comment
Log in with itch.io to leave a comment.